本記事は [個人開発 Advent Calendar 2019]の14日目の記事です。
Python×Kivyというフレームワークを使用して、Windowsアプリを作成しています。
背景
- 紙本を自炊してPDFにした。スッキリ!
- さぁ、iPadで読もう
- 子供にiPad取られた・・・
- Surface買った!さぁ、Surfaceで読もう
- iPadと違ってWindows用の良いアプリ無いな
- Kinoppy(紀伊国屋書店アプリ)良いね!
- これ今勉強中のKivyで作れるかな。
Kinoppy(紀伊国屋書店アプリ)はとても良いアプリで、おすすめです。
アプリ概要
本棚とPDFビューワ機能を持っています。
開発環境
AnacondaNavigator
VSCode
Python3.7
Kivy1.11
使用ライブラリ等
他人に頼りすぎて沢山ありますが、メインで使用しているものは以下です。
PDFレンダリング:pdf2image (https://github.com/Belval/pdf2image)
設定ファイル:ruamel.yaml
アイコンフォント:iconfonts (https://github.com/kivy-garden/garden.iconfonts)
大変お世話になったサイト
Kinoppy (https://k-kinoppy.jp/)
Kivy (https://kivy.org/)
stackoverflow (https://stackoverflow.com/)
荒川光線ブログ 本館 (https://koh-sen.jp/)
そして
Qiita:Kivy (https://qiita.com/tags/kivy)
Todo
結構奥が深くて志半ばですが、以下を目指してます。遠い。。
- ★済★ PDF読込(ローカルPC)
- ☆未☆ PDF読込(DropBox)
- ★済★ 本棚管理(本棚追加&削除)
- ★済★ D&Dで本の移動(本棚内)
- ☆未☆ D&Dで本の移動(別の本棚)
- ★済★ ビューワ機能(1or2ページ表示、右or左開き指定)
- ☆未☆ ビューワ機能(自動目次作成)
- ☆未☆ ビューワ機能(検索)
ソースコード他
以下のKivyのソースコードを2つ抜粋して紹介します。
- Scatterによるサムネイルのドラッグアンドドロップ
- Sleiderの右→左の実現
- (おまけ)本棚の作り方
①Scatterによるサムネイルのドラッグアンドドロップ
サムネイル(本棚に表示される画像)を何秒か掴んだらドラッグアンドドロップします、という事で、画像枠に色を付けます。もしドラッグアンドドロップに入る前に離したら、普通のクリックと見なしてビューワに遷移します。
②Sleiderの右→左の実現
海外のPDFViewerで不便に思うのが縦書き(右→左)に対応していない事です。Kivyのスライダーは左→右(下→上)は実現できますが、右から左の設定がありません。あとスライドしている時にページ数も欲しかったのでこちらは部品化しました。ソースはGithubにアップしてあります。こうやって標準部品をカスタマイズするのかーと勉強になりました。もし良ければソースご覧ください。
③(おまけ)本棚の作り方
ちょうどいい本棚画像が無かったので、作りました。この画像の事です。
作り方はこちらのページにて、プログラムとは関係ありませんし、どこにも需要が無さそうですが。
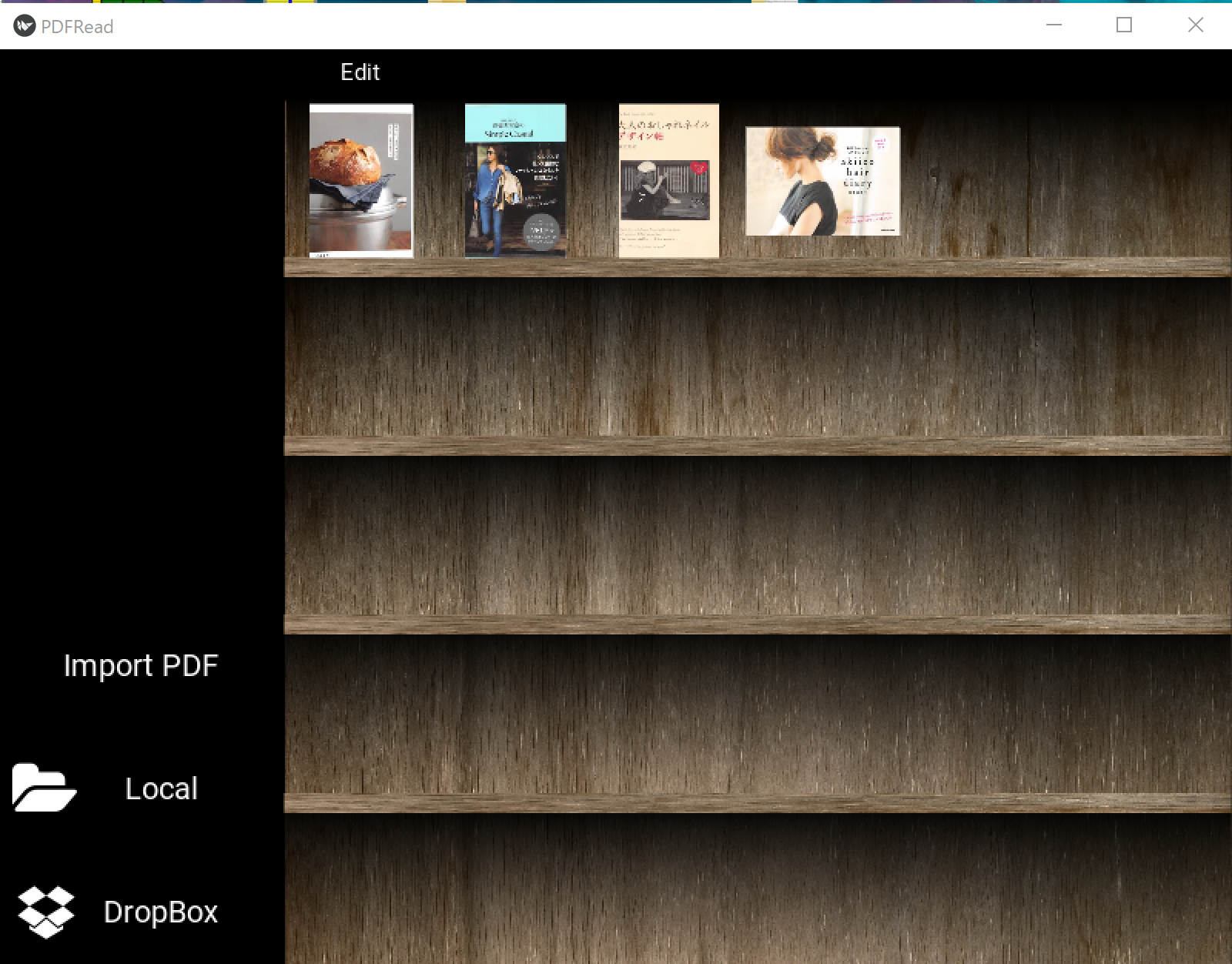
コメント